This page is not created by, affiliated with, or supported by Slack Technologies, Inc.
2023-01-16
Channels
- # announcements (2)
- # babashka (51)
- # beginners (165)
- # biff (39)
- # clara (1)
- # clj-kondo (20)
- # cljsrn (6)
- # clojure (64)
- # clojure-belgium (11)
- # clojure-conj (2)
- # clojure-europe (12)
- # clojure-nl (3)
- # clojure-norway (7)
- # clojure-uk (6)
- # clojurescript (11)
- # conf-proposals (1)
- # conjure (1)
- # core-async (19)
- # cursive (6)
- # data-science (16)
- # datomic (6)
- # deps-new (4)
- # fulcro (60)
- # funcool (3)
- # graalvm (9)
- # helix (14)
- # introduce-yourself (4)
- # jobs-discuss (13)
- # joyride (1)
- # kaocha (2)
- # malli (12)
- # off-topic (25)
- # polylith (9)
- # portal (3)
- # practicalli (1)
- # rdf (43)
- # re-frame (7)
- # reagent (5)
- # releases (5)
- # remote-jobs (8)
- # sci (5)
- # shadow-cljs (42)
- # squint (6)
- # xtdb (5)
Hello! I'm very new to clojure, biff and XTDB, but I'm enjoying every second (despite being stuck on silly stuff sometimes 😅 ) I have a question: how do I manage CORS config in biff?
Welcome! I’m another channel member here who’s happily using Biff and learning a lot thanks to it. Did you run into a CORS error?
A second welcome 🙂 . For CORS you can use https://github.com/r0man/ring-cors, e.g.
(def features
{:api-routes ["/foo" {:middleware [[cors/wrap-cors
:access-control-allow-origin #".*"
:access-control-allow-methods [:get :post]]]}
...]})
You'll probably want to put the routes under :api-routes
instead of :routes
as shown. That'll disable CSRF checks.@U0X9N9ZK5 Thanks! Yes, I was getting No 'Access-Control-Allow-Origin' header
, but solved now 🙂
@U7YNGKDHA thanks! Worked perfectly, just took some time to realize how to add in the deps.edn hehe
Sooo, how do you handle icons? So far I inject SVG elements from http://heroicons.com directly into the code but I'm wondering if there are better ways (I'd rather not introduce a dependency to npm just for this though)
I found this useful: https://v1.tailwindcss.com/course/working-with-svg-icons
But please let me know what you learn/try… I’m at a point where I’m converting placeholder buttons and clickable text to icons myself.
I've been doing it the same way (injecting svg code into the clojure code). Haven't seen that video before, but anything from the tailwind people is probably a good recommendation. My way isn't necessarily the best way, just my way ha ha. I've been using https://fontawesome.com/, and I wrote a helper bb task that takes the pasted svg output from there and formats it as a clojure map + puts it on my clipboard, which I then paste into one of my clj files:
(defn icon []
(let [contents (slurp *in*)
[_ view-box] (re-find #"viewBox=\"([^\"]+)\"" contents)
[_ path] (re-find #"path d=\"([^\"]+)\"" contents)
ret {:view-box view-box :path path}
]
(sh/sh "win32yank.exe" "-i" "--crlf" :in (pr-str ret))))
Used like so:
echo '<PASTED SVG CODE>' | bb icon
Then the clj file looks like this:
(ns com.yakread.ui.icons)
(def data
{"arrow-right" {:view-box "0 0 448 512", :path "..."}
...})
(defn icon [k & [opts]]
(let [{:keys [view-box path]} (data k)]
[:svg.flex-shrink-0.inline
(merge {:xmlns ""
:viewBox view-box}
opts)
[:path {:fill "currentColor"
:d path}]]))
;; example: (icon "arrow-right" {:class "w-5 h-5"})
Nice thing about inserting the svg code directly is it gives you lots of flexibility for styling> But please let me know what you learn/try Will do! Still figuring this shit out 🙂
(This icon stuff might make for a good addition to Biff, or at least a how-to article)
On a related note, the holy grail for me is something that converts HTML snippets to rum… I’ve been using html2hiccup for now (a webpage tool that also has code on GH) which works with a few occasional tweaks.
Not that I would use HTML snippets or anything. Just asking for a friend. 😳
> (This icon stuff might make for a good addition to Biff, or at least a how-to article) eelchat doesn't support emojis, does it? 😛 I really like your approach btw > something that converts HTML snippets to rum 💯 html2hiccup uses hickory underneath, I suppose one idea would be to add it as a dev dependency and use this: https://github.com/buttercloud/html2hiccup/blob/2560629a2492dd0052252d10ba5bfc2b45265413/src/cljs/html2hiccup/core.cljs#L35
htmltohiccup was another one but it doesn't run anymore now that heroku stopped doing their free plans or whatever it was...
Ah yes, I was gonna say you could do something with Hickory. The main problem I've run into is just styles I think--you could do a postwalk
over the as-hiccup
output and convert the :style
strings to maps.
maybe add a bb task for it if hickory works with bb?
How would that work? bb task <input file>?
maybe have it read from stdin
like with the icon task
My sense is that a good workflow may be having a buffer open (emacs guy here) into which I paste the snippet into a def, then use a function to convert it… because sometimes there are errors in the converted output that are best resolved in the html input and that could be done on the spot… in my case I would evaluate the output right in the buffer (using the repl there) and if it throws an error fix the source.
That would open up the possibility, too, of being able to slurp in html from wherever.
But it’s not either/or… the bb approach would be based on something that could be used in the repl, too.
There are beautiful html Tailwind snippet libraries out there which really speed things up.
What port changes do I need to change to run an additional bb dev? I changed what I thought would do the trick but am getting ‘address already in use’ errors.
Is this what you tried to change?
:biff/port 8080
:biff/base-url ""
(in your config.edn)[main] INFO app - Go to
Execution error (BindException) at java.net.PlainSocketImpl/socketBind (PlainSocketImpl.java:-2).
Address already in use (Bind failed)
Maybe it's the nrepl port?
that’s what I’m wondering, too.
I’m not sure how I’d change that, though.
I do that sometimes 🙂
yeah, you'll need to change the webserver port and the nrepl port. :biff/port
does the webserver port. If you've upgraded to the latest version of biff, there's a :biff.tasks/clj-args
config option you can edit. see https://github.com/jacobobryant/biff/releases/tag/v0.5.6

and https://github.com/jacobobryant/biff/commit/750d9897bd5cfe508a7e6d90f9c6bdbc906db750#diff-457a8574a0772bef7ce54f354ba6ce1a73dd8203d548152ed53b517cf136a63e, linked from that release page
alternately, it is also very easy to just hardcode the change. you can change this, from your main clj file:
(defn -main [& args]
(start)
(apply nrepl-cmd/-main args))
to this:
(defn -main [& args]
(start)
(nrepl-cmd/-main "--port" "7889" "--middleware" "[cider.nrepl/cider-middleware,refactor-nrepl.middleware/wrap-refactor]"))
Random thought: maybe I should write the biff newsletter more frequently and just go over stuff that comes up here in slack
Well I didn't manage to write the newsletter this week, so I'll give it a chance next week. mondays are my writing days, though I mainly spend it on the https://tfos.co/. I did write an outline though (probably won't get to it all in one post): > Stuff to write about: > • icons > • running two biff projects > • cors > • 400 stars lol > • safelisting tailwind classes > • refactoringui > • using other databases > • the spectrum of interactivity > • when is biff not the right choice/comparison to other frameworks > • frameworks and libraries > • why I like clojure > • no more meetups--I'm essentializing > roadmap: > • currently working on better secret management > • DO 1-click image > • s3 backup + restore > • better authentication > • something to think about: the DI framework + passing context map around and stuff, is there a better way or not > • then: platypub > • and writing newsletter/docs on mondays
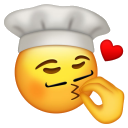