This page is not created by, affiliated with, or supported by Slack Technologies, Inc.
2022-05-04
Channels
- # announcements (4)
- # aws (3)
- # babashka (58)
- # beginners (59)
- # biff (6)
- # cider (3)
- # clj-kondo (48)
- # clj-on-windows (1)
- # cljdoc (1)
- # clojure (136)
- # clojure-europe (19)
- # clojure-gamedev (7)
- # clojure-germany (2)
- # clojure-nl (7)
- # clojure-norway (1)
- # clojure-portugal (1)
- # clojure-uk (4)
- # clojurescript (41)
- # community-development (2)
- # core-async (5)
- # cursive (10)
- # data-oriented-programming (1)
- # data-science (1)
- # datahike (5)
- # datomic (60)
- # docker (2)
- # emacs (13)
- # figwheel-main (19)
- # fulcro (12)
- # graalvm (9)
- # holy-lambda (41)
- # honeysql (14)
- # introduce-yourself (3)
- # jobs (4)
- # lsp (11)
- # nrepl (1)
- # off-topic (9)
- # other-languages (2)
- # pathom (22)
- # portal (5)
- # re-frame (17)
- # remote-jobs (4)
- # reveal (14)
- # shadow-cljs (1)
- # tools-build (7)
- # tools-deps (47)
- # xtdb (8)
- # yada (2)
Hi. How would you get the last ten 10 chars of a string? Calculate the total count ? or do we have something like [-10:] as in Python?
(let [s "abcdefghijklmnop"] (subs s (- (count s) 10)))
Yeah, counting. And the string has to be at least 10 characters long, otherwise you'll get an exception.
Definitely more cumbersome than in Python, yeah. On the other hand, it's easy to write your own function that would work the same way on any data structure you need.
I'd do that only in a REPL. On the other hand, maybe that's exactly what the OP wanted, so good point.
> (apply str (take-last 10 “asdf asdfasdfasdf asdf”)) The above follows into clojures seq philosophy, but is more verbose.
Maybe this is a silly question, but how far can I get with Clojurescript without a good understanding of Javascript? I'm finding that everything is a slog as I try to learn and wonder if it is my lack of knowledge holding me back.
I'm not an expert but maybe a beginner-ish might have a good perspective - I don't know how much software stuff you know in general but it's worth disambiguating: javascript the 'pure' language javascript runtime environments (browser, node, etc), javascript build ecosystem, the browser elements (html, CSS), javascript library ecosystem (react, redux etc)
in my experience, getting into and hacking at the runtime and dealing with the build systems is by far harder than anything related to understanding javascript the language, and the browser is it's own bottomless pit of complexity
so it depends what you're trying to do, just to write data manipulating code or something - you just need to have an understanding of getting your REPL connected to a runtime, and then javascript matter so far as you are using any libraries/interop, and errors
If I want anything outside of a browser, I have a lot of wonderful options that aren't JS.
yeah, tbh it's not my specialism but when I tried to do that a while ago my problem was not knowing about html/react/typescript/webpack much more than not knowing javascript
I think that shows pretty clearly that I haven't been thinking about the problem the right way. I've just lumped all that under "Javascript" in the way you'd have to learn lein/clj for Clojure or cargo for Rust. I can't get a good mental model of how it is supposed to all work together.
this is all subjective but what you're describing is a build system, and if you're in pure cljs then you wouldn't need to know anything other than cljs building but for browser stuff it's very unlikely not to be using react/etc, so at least a passing knowledge of the equivalent of lein which is like yarn/npm/webpack/ts is very useful
but that shits ten times more complicated than most build ecosystems imo as it's been gradually evolved independently in a very cargo-cult fashion in order to torture and degrade javascript devs and ultimately hold back humanity's progression [citation needed]
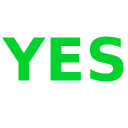
This is where I struggle as well. I found myself having a disproportional understanding of Clojure compared to my relative ineptitude when it comes to the web. The "elephant in the room" seems to be that the web has become ridiculously complicated and IMO being a frontend dev has become a much higher bar than the industry is willing to acknowledge. And as soon as you want to do anything "interesting" such as, say you find a cool library and want to integrate it into your app, or an algorithm (that's in JS) that you'd like to port, you're at least going to have to have a basic fluency. My strategy has been to go through the MDN docs (the https://developer.mozilla.org/en-US/docs/Learn guide is a good start) as if I'm simply learning JavaScript, and hoping that things will start to even out.
in terms of tooling I use calva on vscode and shadow-cljs but I don't know if that's better or worse than other options, it's good to know that you don't have to simultaneously learn emacs, people also recommend cursive I think but not sure whether for cljs or clj specifically
I've done so much Clojure with Vim that the dev tooling doesn't worry me. There are some oddities with Fireplace and the JS-based repls, but I haven't sunk much time into resolving them yet.
if you have circular dependencies in clojurescript and want to use your own forks, how do you achieve that given that deps.edn seems to demand a SHA for git dependencies and these definitionally can't be mutated?
why do you have circular dependencies?
Hi am trying to use the VS code editor with the calva extension and Leinengen for ClojureScript development , but I am having trouble with code navigation , navigating to function definitions fails for me ( VS code transitions to loading and is stuck ) my shadow-clj.edn config
{:lein true
:nrepl {:port 8777}
:builds {:app {:target :browser
:output-dir "resources/public/js/compiled"
:asset-path "/js/compiled"
:modules {:app {:init-fn stellar.awesome-o.core/init
:preloads [devtools.preload
re-frisk.preload
;; day8.re-frame-10x.preload
]
:main {:init-fn stellar.awesome-o.core/init}}}
;; :release {:build-options
;; {:ns-aliases
;; {day8.re-frame.tracing day8.re-frame.tracing-stubs}}}
:closure-defines {"re_frame.trace.trace_enabled_QMARK_" true
;; "day8.re_frame.tracing.trace_enabled_QMARK_" true
stellar.awesome-o.config/base-uri #shadow/env "APP_URL"}
:devtools {:http-root "resources/public"
:http-port 8280
}}}}
Here is my project.clj
(defproject stellar.awesome-o "1.0.0-SNAPSHOT"
:dependencies [[org.clojure/clojure "1.10.1"]
[org.clojure/clojurescript "1.10.597"
:exclusions [com.google.javascript/closure-compiler-unshaded
org.clojure/google-closure-library
org.clojure/google-closure-library-third-party]]
[thheller/shadow-cljs "2.8.83"]
[reagent "0.10.0"]
[re-frame "0.11.0"]
[day8.re-frame/http-fx "v0.2.0"]
[hickory "0.7.1"]
[garden "1.3.9"]
[ns-tracker "0.4.0"]
[com.taoensso/timbre "4.10.0"]
[day8.re-frame/http-fx "v0.2.0"]
[reagent-utils "0.3.3"]
[mattinieminen/re-fill "0.2.0"]
[re-pollsive "0.1.0"]]
:plugins [[lein-garden "0.3.0"]
[lein-shell "0.5.0"]]
:min-lein-version "2.5.3"
:jvm-opts ["-Xmx1G"]
:source-paths ["src/clj" "src/cljs"]
:clean-targets ^{:protect false} ["resources/public/js/compiled" "target"
"resources/public/css"]
:garden {:builds [{:id "screen"
:source-paths ["src/clj"]
:stylesheet stellar.awesome-o.css/screen
:compiler {:output-to "resources/public/css/screen.css"
:pretty-print? true}}]}
:shell {:commands {"open" {:windows ["cmd" "/c" "start"]
:macosx "open"
:linux "xdg-open"}}}
:aliases {"dev" ["with-profile" "dev" "do"
["run" "-m" "shadow.cljs.devtools.cli" "watch" "app"]]
"prod" ["with-profile" "prod" "do"
["run" "-m" "shadow.cljs.devtools.cli" "release" "app"]]
"build-report" ["with-profile" "prod" "do"
["run" "-m" "shadow.cljs.devtools.cli" "run" "shadow.cljs.build-report" "app" "target/build-report.html"]
["shell" "open" "target/build-report.html"]]
"karma" ["with-profile" "prod" "do"
["run" "-m" "shadow.cljs.devtools.cli" "compile" "karma-test"]
["shell" "karma" "start" "--single-run" "--reporters" "junit,dots"]]}
:profiles
{:dev {:dependencies [
[re-frisk "1.3.2"]
[binaryage/devtools "1.0.0"]]
:source-paths ["dev"]}
}
:prep-tasks [["garden" "once"]])
also I keep getting this error when running Leinengen + Shadow CLJs ; Error while connecting cljs REPL: TypeError: Cannot read properties of undefined (reading 'search')
@zimablue even so I don't think Closure even supports that so you're going to bottom out there?
Supports circular project references? Maybe I'm going mad but previously I had thought that this project contained them and compiled, working from my notes, I will check and get back
As in compiled when either everything was local or clojars, not specified as git dependencies
you can Google for this, it appears to be something that should generally be avoided, goog.module
has some support but we're never going to support that because it breaks the REPL