This page is not created by, affiliated with, or supported by Slack Technologies, Inc.
2019-11-27
Channels
- # announcements (2)
- # babashka (60)
- # beginners (73)
- # calva (23)
- # cider (2)
- # clj-kondo (19)
- # cljs-dev (31)
- # clojure (29)
- # clojure-berlin (1)
- # clojure-europe (6)
- # clojure-nl (17)
- # clojure-spec (21)
- # clojure-uk (15)
- # clojurescript (54)
- # core-async (48)
- # cursive (35)
- # datomic (12)
- # emacs (12)
- # fulcro (66)
- # graalvm (3)
- # graphql (16)
- # jackdaw (1)
- # malli (1)
- # off-topic (11)
- # pedestal (4)
- # re-frame (10)
- # reitit (1)
- # rewrite-clj (8)
- # ring-swagger (8)
- # shadow-cljs (14)
- # spacemacs (2)
- # vim (5)
Is there a way to invoke a constructor in CLJS with an array instead of specifying the args separately? e.g.
(new* js/Error ["hello"])
apply
works because Error
can be called as a function and still behave as a constructor. this is true of many constructors in JavaScript, but not everyone
@christian767 can you give an example when this would not work? and what would be the proper solution in general?
function Constructor(a, b, c) {
if (!(this instanceof Constructor)) {
return new Constructor(a, b, c);
}
// ...
}
@borkdude it will work with any constructor that does this ^^but not all of them do. for instance String(...)
behaves differently from new String(...)
. With user provided constructors there's no way to tell other than checking the source.
oh ok, but is there is a way to invoke new from cljs with an array of args instead of single ones?
new Error("message")
// is equivalent to
var errorInstance = Object.create(Error.prototype);
var error;
error = Error.call(errorInstance, "message")
apply should work, just tested it with Error
error = Error.apply(errorInstance, ["message"])
cool, seems to work indeed:
(defn invoke-constructor #?(:clj [_ctx ^Class class args]
:cljs [_ctx class args])
#?(:clj (Reflector/invokeConstructor class (object-array args))
:cljs (let [args (into-array args)
obj (js/Object.create (.-prototype class))
obj (.apply class obj args)]
obj)))
awesome!
Hi, I am getting something very strange and wonder whether someone can hint to what is going on. The context is a fulcro app in the browser so it could be related to react or something. Here I map over notes, which works:
(dom/div (map ui-note (sort :note/id notes)))
ui-note is a factory for a react component.
It works but it sorts differently in chrome and firefox. In firefox it ignores the sort, in chrome it sorts fine.
but
(dom/div (map ui-note (sort #(> (:note/id %1) (:note/id %2)) notes)))
and
(dom/div (map ui-note (sort #(> (:note/id %1) (:note/id %2)) notes)))
work the as expected in both firefox and chrome.
This behavior is there in development builds and production builds. I use shadow-cljs. It is not urgent, since it works with the lambda version but it confuses the heck out of me.
Can anybody point me in a direction? I do not know how to google this.
Thanks in advance!!Can you remove the dom related stuff and reproduce the bad sort in the repl with just data?
Its not the repl, but I changed it to (str (sort :note/id notes)) and they sort differently.
Ok. I have a webpage that renders
(str (sort :note/id '({:note/id 1} {:note/id 2}))).
Result on chromium 76.0.3809.132 freebsd and same on android:
({:note/id 1} {:note/id 2})
Result on firefox 70.0 on freebsd:
({:note/id 2} {:note/id 1})
Clojurescript Version as of deps.edn: 1.10.520I can repro this as well
@magra you are using it wrong, sort takes comparator fn which takes two args
(str (sort #(compare (:note/id %1) (:note/id %2)) '({:note/id 1} {:note/id 2})))
I think in your case itβs undefined behavior
Make sure we are thoroughly represented here, people: https://survey.stateofjs.com/ π
you will the same result in jvm clojure, the problem is floating point numbers
Here's a useful site about this https://floating-point-gui.de/
Something like this decimal library might help: https://funcool.github.io/decimal/latest/
https://stackoverflow.com/questions/11695618/dealing-with-float-precision-in-javascript
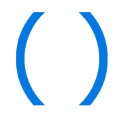
Hey there, I was trying to use cemerick's fork of valip in a project, but when I try to start the server it throws Syntax error (IllegalAccessError) compiling at (valip/predicates.clj:1:1).read-string does not exist
Do I have to include cljs on the server side somehow?
Nevermind, figured out how .cljc works
Hey guys, trying shadow for the first time (figwheel-main was my go to so far) .. starting up via cider M-x cider-jack-in-cljs gives me a clojure.lang.ExceptionInfo : missing instance { } error ... any clue what this is about?
I'm guessing its looking for a shadow server. And lein should have started it but hasn't managed to?
Has anyone worked with Google Maps Javascript API (on client side) with shadow cljs? Assuming I load the library with my API key through the html page, how do I then create a Map object to configure it?
solved: Run via shadow-cljs not lein in the cider popup. guessing it was a no-go on the build triggering server/start!. Suggest adding this to the user manual at https://shadow-cljs.github.io/docs/UsersGuide.html#cider
it is specified here:
> CIDER will prompt you for the type of ClojureScript REPL:
>
> Select ClojureScript REPL type:
>
> Enter shadow.
This refers to the second popup which asks to select between different REPLs - figwheel, nashorn, shadow etc This is taken care of in the .dir-locals.el file
Let me get done with work, and ill remove the dir-locals and screenshot the 3 popups that come up in series. First popup: choose build tool : lein or shadow-cljs Second: choose REPL: figwheel, shadow, nashorn etc Third: choose shadow build: app, test etc
Can share emacs/cider versions if it helps, and if this is not behaviour that is consistently observed.
no that's the correct steps. it needs to know which executable to use to run, then what type of cljs repl you want, and then which build
thanks @U11BV7MTK.. do you know how i can contrib to the docs, can't find it on the repo
for CIDER just send a PR to the docs https://github.com/clojure-emacs/cider/tree/master/doc/modules/ROOT/pages/basics