This page is not created by, affiliated with, or supported by Slack Technologies, Inc.
2022-06-04
Channels
- # announcements (3)
- # aws (13)
- # babashka (10)
- # beginners (30)
- # biff (1)
- # calva (59)
- # chlorine-clover (11)
- # cider (3)
- # circleci (2)
- # clojars (22)
- # clojure (21)
- # clojure-europe (2)
- # clojurescript (10)
- # core-typed (2)
- # fulcro (8)
- # girouette (12)
- # graphql (1)
- # helix (6)
- # inf-clojure (4)
- # joyride (6)
- # leiningen (7)
- # off-topic (3)
- # pathom (44)
- # polylith (13)
- # shadow-cljs (44)
- # tools-deps (1)
I install java with asdf
. I am constantly seeing this warning, which is a bit annoying though:
Warning: environ value /Users/pinkfrog/.asdf/installs/java/zulu-17.30.15 for key :java-home has been overwritten with /Users/pinkfrog/.asdf/installs/java/zulu-17.30.15/zulu-17.jdk/Contents/Home
It comes from this library: https://github.com/weavejester/environ
And it complains that your JAVA_HOME
environment variable does not point to the Java that you're actually running.
I've seen that warning in one of the projects I'm working on. Just searched the contents of its classpath.
I don't, I don't really care. If you do, just set JAVA_HOME
to the right value when starting Java.
Does Clojure have a function that acts like ignore
which takes a variadic number of arguments and does nothing?
Anyone familiar with leiningen and setting a clojure version outside of a project? https://clojurians.slack.com/archives/C0AB48493/p1654334489881789
Hi guys, I’d like to know the idiomatic way of writing the following function
(defn- path-to-ns
[path]
;; "src/foo/bar" -> "foo.bar.main"
(let [path (str path "/main")
idx (inc (str/index-of path "/"))
path (subs path idx)
path (str/replace path #"/|-" {"/" "."
"-" "_"})]
path))
(path-to-ns "src/foo/bar")
(defn- path-to-ns
[path]
;; "src/foo/bar" -> "foo.bar.main"
(let [path (str path "/main")
idx (inc (str/index-of path "/"))]
(-> (subs path idx)
(str/replace #"/|-" {"/" "."
"-" "_"}))
(path-to-ns "src/foo/bar")
Sorry about formatting, I think this is marginally better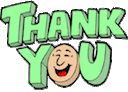
My attempt:
(defn path-to-ns [path]
(->> (-> (str/replace path #"-" "_")
(str/split #"/")
(conj "main")
rest)
(str/join ".")))
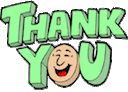
Found something curious today, pop
works for vector and list but it doesn't work for java.lang.Cons
or clojure.lang.LazySeq
. Is this by design? I guess it makes sense if it's by design since pop is supposed to work on "closed" data structures as opposed to "open" things that can return an infinite number of elements like lazy seqs, etc.
user=> (pop [1 2 3])
[1 2]
user=> (pop '(1 2 3))
(2 3)
user=> (pop (cons 1 '(2 3)))
Execution error (ClassCastException) at user/eval5 (REPL:1).
class clojure.lang.Cons cannot be cast to class clojure.lang.IPersistentStack (clojure.lang.Cons and clojure.lang.IPersistentStack are in unnamed module of loader 'app')
user=> (pop (lazy-seq (cons 1 (lazy-seq nil))))
Execution error (ClassCastException) at user/eval7 (REPL:1).
class clojure.lang.LazySeq cannot be cast to class clojure.lang.IPersistentStack (clojure.lang.LazySeq and clojure.lang.IPersistentStack are in unnamed module of loader 'app')
Thanks, I think sometimes we conflate those things with lists due to how they operate similarly in some aspects, or how similar they look as outputs.
yeah, I get it. you should remember that all those things are seqs, not necessarily lists 🙂
I suspect this is because a cons or lazy seq can go over a collection that peek/pops differently right? You’re “breaking” the data structure
(peek [1 2 3])
-> 3
(peek (list 1 2 3))
-> 1
so if you could (peek (cons 0 [1 2 3]))
you’ve created a mismatch and scrambled your queue/stack. The cons would add the 0
at the “wrong” end