This page is not created by, affiliated with, or supported by Slack Technologies, Inc.
2023-06-07
Channels
- # aleph (5)
- # announcements (2)
- # babashka (17)
- # beginners (13)
- # cider (4)
- # clj-kondo (9)
- # cljdoc (18)
- # clojure (20)
- # clojure-art (7)
- # clojure-dev (11)
- # clojure-europe (20)
- # clojure-nl (2)
- # clojure-norway (53)
- # clojure-spec (30)
- # clojure-uk (4)
- # clojurescript (40)
- # datomic (70)
- # events (2)
- # graalvm-mobile (8)
- # gratitude (2)
- # guix (2)
- # honeysql (3)
- # hyperfiddle (10)
- # introduce-yourself (4)
- # jobs (2)
- # lsp (6)
- # luminus (3)
- # malli (5)
- # polylith (35)
- # practicalli (6)
- # re-frame (3)
- # reagent (9)
- # releases (1)
- # remote-jobs (20)
- # ring-swagger (2)
- # shadow-cljs (12)
- # sql (18)
- # xtdb (7)
I naively thought I could do this to get some JSON from a shell command, and parse it:
(ns leifs-utils.core
(:require [babashka.process :as process]
[cheshire.core :as json]))
(defn get-projects [org-url]
(-> (process/shell (str "az devops project list --org " org-url))
(json/parse-string true)))
(process/shell "az devops project list --org ")
But that will result in the following error:
java.lang.ClassCastException: babashka.process.Process cannot be cast to java.lang.String
How can I get- and parse the JSON from the shell command, instead of outputting it in the terminal?(-> (process/shell {:out :string} (str "az devops project list --org " org-url))
:out
(json/parse-string true))
I thought maybe so, and tried that earlier, but then I got this error instead:
java.lang.ClassCastException: java.lang.ProcessBuilder$NullInputStream cannot be cast to java.lang.String
Here is the whole code snippet:
(ns leifs-utils.core
(:require [babashka.process :as process]
[cheshire.core :as json]))
(defn get-projects [org-url]
(-> (process/shell (str "az devops project list --org " org-url))
:out
(json/parse-string true)))
(get-projects " ")
btw, you don't have to concatenate org-url with a space, just provide it as another argument:
(process/shell {:out :string} "az devops project list --org" org-url)
If you (-> (sh "foo") :out ...)
it should "just work"
It's babashka.process/sh
You can see it in my repo, here:
https://github.com/leifericf/leifs-utils/blob/main/src/leifs_utils/shell.clj
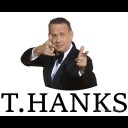
How do I run a -m
main function from a task alongside :extra-paths
?
clj -M:your-alias -m AClass
?or you could put :main-opts into an alias
i mean in babashka, lol